In IDEs from JetBrains you can mark strings as being from another language. This is for example useful for regular expressions, SQL (together with the "Database Tools and SQL" plugin), or strings containing HTML. This also works for JavaScript snippets embedded in your code.
For example, in the following snippet I can instruct the IDE to let the string be recognised as SQL:
let sql = "SELECT * FROM users WHERE id = 1;";
Example snippet for using the IDE's language injection feature with SQL.
You can either open the context menu and use the menu entry "Show Context Actions" or use the associated keyboard shortcut you have configured to open the "Context Actions" menu directly:

In this new menu, you can select "Inject language or reference" as follows:

Afterwards, the IDE presents you with a list of possible language to use. You can either manually select your language or start typing to search through the list. This search works in most of the IDE's menus, by the way.
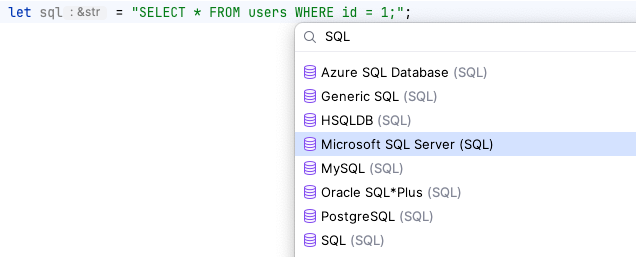
By using language injection you can use all the features the IDE provides for that injected language. For the example above, with a configured data source, the IDE now informs me that my query refers to a non-existent table:

The problem is, that this injection is temporary and disappears after you close the file. For some time I did not check the official documentation on the language injection feature and also did not use the feature much. There are actually several ways to make it more-or-less automatic.
In the following snippet, I'm using the wasm_bindgen
crate in Rust to configure some bindings to JavaScript. It also supports inlining JS snippets. By adding a comment indicating the language I can instruct the IDE to permanently use language injection:
// language=JavaScript
#[wasm_bindgen(inline_js = r#"
import { App } from "realm-web";
export async function run() {
// ...
App.getApp('my-app-id');
// ...
}"#)]
extern "C" {
#[wasm_bindgen]
async fn run();
}
Example snippet for using language injection with a magic comment.
As before, the IDE provides support for the injected language. As I have a package.json
with the realm-web
library included and used npm i
to install the dependencies, I get auto-completion when using the package:
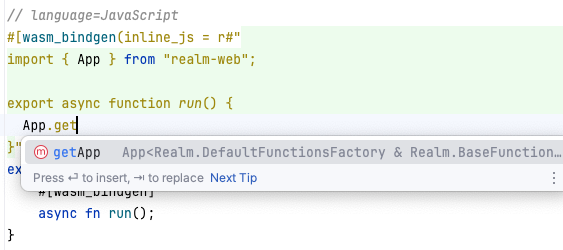
The syntax highlighting is kind of wonky for JavaScript in Rust's raw string literal using RustRover. You can also edit the fragment in isolation in a separate editor tab:
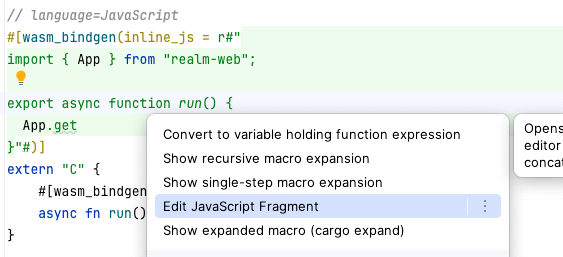
Although in that separate tab, the auto-completion is not working well but the syntax highlighting is better. Please note, that I'm using an IDE fresh out of beta (RustRover) in a non-standard project (Cloudflare Worker with Rust via WASM) which may affect how well it works.
There are also automatic rules you can configure for detecting languages in your string based on how they are used or you can also use annotations in Java. Please refer to the official documentation on the language injection feature for more details.
Language Injection in JetBrains IDEs
A valuable feature in JetBrains IDEs allows interpreting a string as a different programming language, such as SQL, with auto-completion support. Additionally, you can use comments to mark strings as being from another language.